Simple Calculator Application using java swing
Design Parts:
- Open NetBeans IDEs.
- Go to File menu à New Project .
- On click on New Project a new Dialog box will come, Select Categories : java and Projects : java Application, Click on Next Button.
- A new Dialog box will appear, Write your Project name and then click on Finish Button. Project is Created.
- Now Right click on your Project, Go to New àJFrame Form.
- A new page will open, we need to design this Form from Palette by Drag and Drop.
- Drag TextField from palette and Drop on JFrame, Set background and foreground color to black and white respectively.
- Drag Button from palette and Drop on JFrame, and Copy Same Button and Paste it 18 times in JFrame.
- Rename All Buttons as ( 0 to 9),(.),(/,X,+,-) and (c,<-,=).
Note: Set Property of all Button and JFrame as per your Choice.
Programming Parts:
1) Define the Variables in the className as follows:
{`public className calciForm extends javax.swing.JFrame { String str=""; int opera =0,id =0; double value1=0,value2=0; public calciForm() { initComponents(); display.setText("0"); } }`}
2) On double click on any numeric Button, Source Code Page will open and Cursor will blink near Numeric Button. Paste the below code between open and close bracket according to numeric Button.
{` For Button Zero:- private void zeroMouseClicked(java.awt.event.MouseEvent evt) { if(!str.equals("")) { str = str +"0"; display.setText(str); } else { str=""; display.setText("0"); } } For Button 1:- private void oneMouseClicked(java.awt.event.MouseEvent evt) { str = str +"1"; display.setText(str); } For Button 2:- private void twoMouseClicked(java.awt.event.MouseEvent evt) { str = str +"2"; display.setText(str); } For Button 3:- private void threeMouseClicked(java.awt.event.MouseEvent evt) { str = str +"3"; display.setText(str); } For Button 4:- private void fourMouseClicked(java.awt.event.MouseEvent evt) { str = str +"4"; display.setText(str); } For Button 5:- private void fiveMouseClicked(java.awt.event.MouseEvent evt) { str = str +"5"; display.setText(str); } For Button 6:- private void sixMouseClicked(java.awt.event.MouseEvent evt) { str = str +"6"; display.setText(str); } For Button 7:- private void sevenMouseClicked(java.awt.event.MouseEvent evt) { str = str +"7"; display.setText(str); } For Button 8:- private void eightMouseClicked(java.awt.event.MouseEvent evt) { str = str +"8"; display.setText(str); } For Button 9:- private void nineMouseClicked(java.awt.event.MouseEvent evt) { str = str +"9"; display.setText(str); } `}
3) On double click on Decimal Button, Source Code Page will open and Cursor will blink near Numeric Button. Paste the below code between open and close bracket :
{` private void decMouseClicked(java.awt.event.MouseEvent evt) { str = str +"."; display.setText(str); }`}
4) On double click on (/,x,+,-) Button, Source Code Page will open and Cursor will blink near Numeric Button. Paste the below code between open and close bracket according to arithmetic operation:
{` For Button ÷ :- private void divMouseClicked(java.awt.event.MouseEvent evt) { value1 = Double.parseDouble(str); opera =1; str = str + "/"; int n = str.length(); id = n; display.setText(str); } For Button X :- private void mulMouseClicked(java.awt.event.MouseEvent evt) { value1 = Double.parseDouble(str); opera =2; str = str + "X"; int n = str.length(); id = n; display.setText(str); } For Buttton + :- private void addMouseClicked(java.awt.event.MouseEvent evt) { value1 = Double.parseDouble(str); opera =3; str = str + "+"; int n = str.length(); id = n; display.setText(str); } For Button - :- private void subMouseClicked(java.awt.event.MouseEvent evt) { value1 = Double.parseDouble(str); opera =4; str = str + "-"; int n = str.length(); id = n; display.setText(str); }`}
5) On double click on (<-,C,=) Button, Source Code Page will open and Cursor will blink near Numeric Button. Paste the below code between open and close bracket:
{` For Button <- : - private void delMouseClicked(java.awt.event.MouseEvent evt) { // TODO add your handling code here: str = str.substring(0,str.length()-1); display.setText(str); } For Button C :- private void clearMouseClicked(java.awt.event.MouseEvent evt) { // TODO add your handling code here: str =""; display.setText("0"); } For Button = :- private void equalMouseClicked(java.awt.event.MouseEvent evt) { // TODO add your handling code here: String str1 = str.substring(id,str.length()); value2 = Double.parseDouble(str1); double res=0; switch(opera) { case 1: res = value1/value2; break; case 2: res = value1*value2; break; case 3: res = value1+value2; break; case 4: res = value1-value2; break; } str = String.valueOf(res); display.setText(str); // str=""; }`}
Output :-
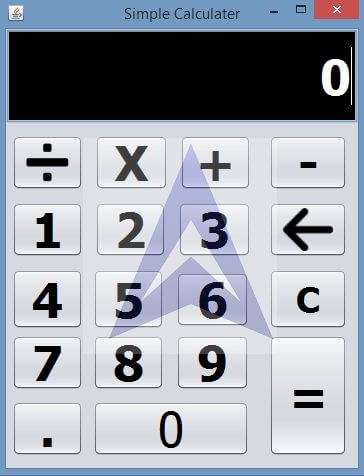