Word Guessing Game Using HTML, CSS And JavaScript
In this assessment, you are to create a word guessing game. The game selects a secret word and displays the letters in the word as dashes. For example, if the secret word was “APPLE”, the game would display the word as _ _ _ _ _.
The player wins if they guess all the letters in the word. They lose if they run out chances before the entire word is completed.
The player uses the game board to choose a letter. If they picked a letter that is in the word, the appropriate dashes in the word are replaced with the selected letter. For example, if the secret word is APPLE, and the user selects the letter “P”, the dashes will be updated to be: _ P P _ _. Next, if they select the letter “E”, the dashes will update to say: _ P P _ E. This process continues until either all correct letters are selected; or, the player runs out of chances.
Once a letter is selected, it cannot be selected again.
TASK
- Develop the user interface for the game using HTML/CSS. Your UI should display:
- A button for starting a new game.
- The secret word, as dashes
- The gameboard where the player can select individual letters
- The number of chances remaining
- Develop the Javascript code to implement the logic of the game.
Submission Instructions
Carefully review the following submission checklist:
- Submit a zip file containing your code to the course dropbox. Your submission must include all the HTML, CSS, and JS files required to run the app. No 7z or rar files accepted.
- Name your file a3-studentname-studentid.zip. Replace studentname and studentid with your name and id.
TECHNICAL REQUIREMENTS
- All code must be written in HTML, CSS, and Javascript. No CSS frameworks or Javascript libraries permitted.
- CSS must be specified in an external style sheet.
- You must use ES6 style Javascript. This means usage of let/const variables, arrow function syntax, etc.
- DOM manipulation should be performed per the practices shown in class, ie: document.querySelector(...) and document.addEventListener(....)
FUNCTIONAL REQUIREMENTS:
- The secret word must be defined in your Javascript code,
- The secret word must be minimum 5 letters long
- The secret word must be an English language word
- When the user taps the START GAME button, the application should update the user interface to display the secret word as dashes. For example, if the selected word is BANANA, then the user interface should display _ _ _ _ _ _.
- The user interface must include a game board. The game board consists of all the letters in the English alphabet. The user guesses a letter by clicking on a letter in the game board. The code for the gameboard is provided in the starter code. You must use the provided code, and you are NOT permitted to modify the provided HTML. You may NOT add classes, ids, or other elements.
- The user can click on any letter. You are not permitted to attach individual event listeners to each letter.
- A letter can only be clicked once. After the letter is clicked, the user interface must change the visual appearance of the letter so that the player knows they cannot click it again.
Example of changing the visual appearance:
- In the screenshot below, the letters A and C have already been selected. The UI updates the letters so they are “greyed out” and non-clickable.
- If the user selects a letter that is in the secret word, update the user interface with the letter in its correct position. For example, if the secret word is BANANA and the user selects the letter “A”, then update the UI to show: _ A _ A _ A
- If the user selects an incorrect letter:
- Display an alert box that notifies the user the letter is not correct. An alert box can be displayed using this code: alert("Hello world!")
- Reduce the number of chances remaining, and update the user interface with the remaining number of chances.
- When the user selects the final correct letter of the secret word, display the secret word (with no dashes) and a “You Win!” message.
- When the user runs out of chances, display the secret word and a “You Lose!” message.
- The user can “restart” the game any time by pressing the “START NEW GAME” button. When the button is pressed:
- Reset the total number of chances
- Reset the secret word to dashes
- Reset the game board so all letters are selectable.
USER INTERFACE
The following screenshots are examples of what the user interface should look like at various stages of the game. You may customize the color and layout.
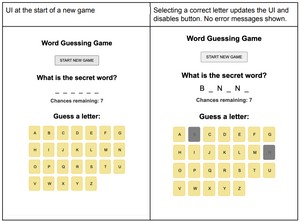
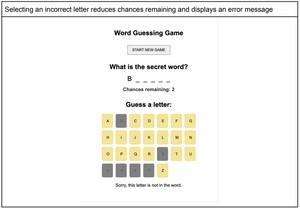
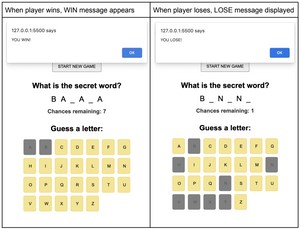
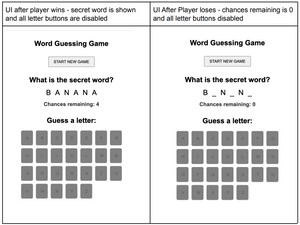