SFDV2104 application software development
Introduction
The project that you will be working on in this course is an online shopping system for Books.
In part 1 you will be creating the administration part of the system that allows the shop staff to add new categories and books to the system.
In part 2 you will be creating the web based user interface that the customers will use to purchase books from the shop.
You will be working on the project throughout the entire course. Each week there will be a project task that is related to the topic covered in the lab that week. By completing each project task each week you will eventually end up with a complete project.
We strongly recommend that you keep on top of the project tasks and don’t fall behind. This is not a trivial project, and you will not be able to leave it until the last minute and complete it.
1 Phase 1
1.1 Requirements Analysis
The requirements analysis identified eight use cases that need to be implemented in this phase of the project:
- Add a new category. (A book can belong to one or more category such as IT, Engineering, Religion, Arabic, Travel etc)
- Viewing all categories.
- Deleting an existing category.
- Editing the details of an existing category.
- Add a new product and associating it with an existing category.
- Viewing all books.
- Deleting an existing book.
- Editing the details of an existing product.
1.2 System Analysis
We have already performed the analysis and design phase of the development. The following is some of the design documentation that describes the system.
Prototype of the GUI
The following are screenshots of the high fidelity prototype:
The Main Menu. This could be a button based menu (as shown) or a standard pull down menu with the company’s logo in the centre of the frame.
The books category editor.
Finding existing categories. This frame is shown when the staff member clicks the “Find” button in the Category Editor.
Deleting a book category. This is shown when a staff member selects a category and clicks the “Delete” button.
Editing an existing category. This is shown when the staff member selects a category and clicks the “Edit” button. The selected category will be loaded into the Category Editor frame so that its details can be edited.
The books editor. Book Categories that are in the system are displayed in a drop down list (JComboBox). The staff member chooses the category that the product they are entering belongs to.
Finding existing products. This frame is shown when the staff member clicks the “Find” button in the Product Editor.
Deleting a product. This is shown when a staff member selects a product and clicks the “Delete” button.
Editing an existing product. This is shown when the staff member selects a product and clicks the “Edit” button. The selected category will be loaded into the Product Editor frame so that its details can be edited.
NOTE: The final implementation of this system doesn’t have to be identical to these screen shots, but should have similar functionality.
System Architecture
The system should be separated into four packages:
- The “domain” package that contains the classes relating to the object-oriented domain model of the business (see the section 1.2 for details about the domain model).
- The “GUI” package that contains the user interface classes for the staff administration GUI. The user interface classes should be created using Swing. The “data access” package that contains the classes that are responsible for storing and finding and otherwise managing the domain objects (products, categories, etc.) that have been entered into the system. To develop Database use MySQL.
- The “web” package that will contain the classes associated with the web based user interface that will be created in the second phase of this project.
The architecture for this system is a logical 3-tier system (as opposed to a physical 3tier system where each tier is separated by a network):
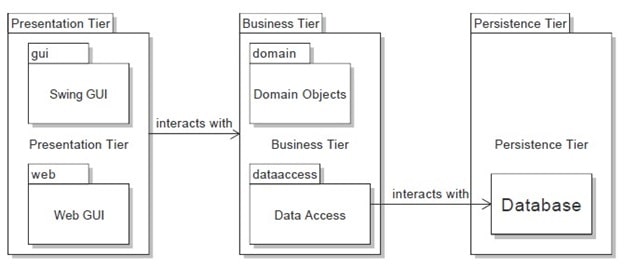
The 3 tiers
The presentation tier provides the user interfaces, the business tier provides the domain objects and the classes that manage them (the data access objects), and the persistent storage tier which in the case of this project is a database provided by a DBMS called MySQL.
Using this architecture gives us better control over the dependencies between the different packages:
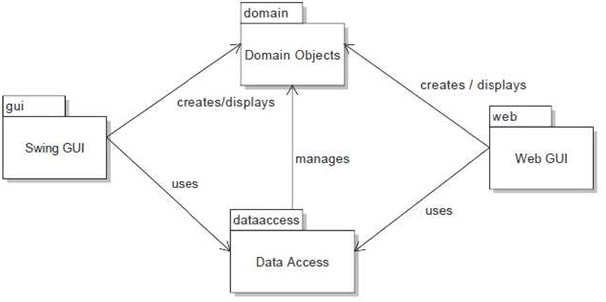
The dependencies between the packages.
Object-Oriented Domain Model
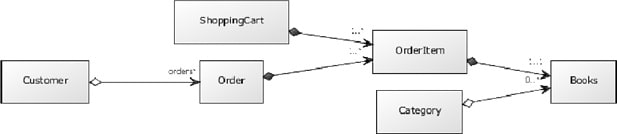
(URL to modify the above UML http://yuml.me/43afef56)
Class Diagram of Domain Model
Book - Contains information about a product that is offered for sale at the shop. Category - Used to group related or similar products to make it easier for customers to find a particular product.
Customer - Contains the account details for a customer of the shop.
Order - Contains information about a single transaction made at the shop.
OrderItem - Represents how much of a single product was purchased in an order.
ShoppingCart - Used to keep track of which products a customer has selected while they are browsing the web site. Once the customer is finished browsing they will “check out” the shopping cart which will create an order using the contents of the shopping cart.
Book, Category, Order, OrderItem, and Customer objects will all be stored in the database. ShoppingCart objects are only temporary, so are not going to be stored.
1.3 Phase 1 Requirements
For this version of the system you are required to:
- Create all of the domain classes shown in section 1.2.
- Create data access classes for the product and category domain objects.
- Create GUIs that allow the user to perform the eight use cases described in section 1.1. 4. Use MySQL for storing product and category objects in the database and for performing all persistence related operations.
2 Phase 2
In this phase you are adding a dynamic web site to the system that will allow your customers to browse and purchase the products that have been entered into the database.
You will be using a web framework using JSP and Servlets 2.1 Use cases for the web site:
- A new customer should be able to create a new account. The customer’s details (as shown in the class diagram in section 1.2) should be stored in the database.
- An existing customer (one who has already created an account) needs to be able to login using the usercode and password that they entered when they created their account.
- The customer needs to be able to view all of the categories that have been stored in the database, and select one for viewing.
- Once the customer has selected a category all products in that category should be displayed, and the customer can select one of them to purchase.
- Once the customer has selected a product all of its details should be displayed, and the customer should be able to enter the quantity of this product that they want to purchase, and add the product to the shopping cart.
- The customer needs to be able to view the contents of the shopping cart including the total cost.
- The customer needs to be able to ‘check out’ the shopping cart. This will cause an order object to be created and added to the database. The quantity on hand values in the products that were purchased should be decremented by the amount that the user purchased. The shopping cart object should be cleared out once the transaction is complete in case the user wants to carry on shopping.
- Finally the customer needs to be able to log out. This should clear any session data that was associated with this customer.
- After logging in the user should always be able to see links that will take them to the categories page, the shopping cart view, or the logout page.
- All dynamic web pages should be controlled by JSP and Servlet
- Add database access should be done via MySQL.