Programming assignment question 2
OBJECTIVES:
Doing this project, students will have the chances to practice with the following concepts; therefore, you should review them when you are working on the project:
- Repetition structures: using for, while, or do..while loop
- Selection structure: using if, if else, or switch statement
- Practice using the array of int, double or string
- Read data from keyboard
- Read data from the file
- Write data to the file
- Using manipulators: setw, fixed, showpoint, or precision, etc. to display data as format
- Using user-defined functions
- How to declare an array and how to initialize or access to array elements
REQUIREMENT:
A store that sales a product that is in either model T224 or model T334.
At the end of each year, the store has the report named Model_T_Sale_yyyy.txt where yyyy is 4 digits of the year, for example, Model_T_Sale_2014.txt or Model_T_Sale_2013.txt, etc. The format of input file should be:
For example: Year 2013 the store has file Model_T_Sale_2013.txt as follows:
{` Jan 2777 1.99 3223 2.19 Feb 2431 1.99 2612 2.19 Mar 2540 1.99 2729 2.19 Apr 2557 2.19 2892 2.29 May 2515 2.19 2249 2.29 Jun 2639 2.19 3190 2.29 Jul 2610 1.89 2839 2.09 Aug 2595 1.89 2875 2.09 Sep 2456 1.89 2828 2.09 Oct 2454 1.99 2803 2.19 Nov 2707 1.99 3295 2.19 Dec 2893 1.99 3522 2.19 `}
For example: Year 2014 the store has file Model_T_Sale_2014.txt as follows:
{` Jan 3128 1.59 3421 1.79 Feb 3130 1.59 2822 1.79 Mar 3126 1.59 3210 1.79 Apr 3075 1.69 3143 1.79 May 3250 1.69 2538 1.79 Jun 3133 1.69 3458 1.79 Jul 3211 1.79 3135 1.89 Aug 2958 1.79 3620 1.89 Sep 2823 1.79 3714 1.89 Oct 2989 1.89 3689 1.99 Nov 3088 1.89 4163 1.99 Dec 2906 1.89 4314 1.99 `}
Where each line has 5 information of month, T224 units sold, T224 unit price, T334 units sold, and T334 unit price.
These files are saved in the storage
This project asks you to provide the pseudo-code and the Java code for the application that processes two tasks:
- Summary Sale in one year
- Sale Comparison between two years
The application continue allows users to use the program until they want to exit
Task1
-Asks users to enter 4 digits for the year in yyyy format, then open the input file Model_T_Sale_yyyy.txt.
-When reading the input file for each line:
*split the line into 4 pieces of the information: month, T224_Units, T224_uPrice, T334_Units and T334_uPrice.
*calculate the monthly sold each model by:
month_T224 = T224 units sold * T224 unit price
month_T334 = T334 units sold * T334 unit price
*calculate the sum of money sold in the year each model:
year_T224 = year_T224 + month_T224
year_T334 = year_T334 + month_T334
*display output in the following format on screen and also write to the output file named SaleSummary_yourLastName_yyyy.txt where yyyy is 4 digits of the year
For example: the output of the summary of sale in year 2013 and the output of the summary of sale in year 2014
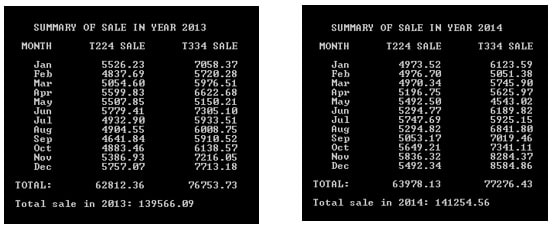
Task 2
-Asks user to enter 4 digits of the first year and enter 4 digits of the second year to compare
-Open 2 input files, Model_T_Sale_xxxx.txt and Model_T_Sale_yyyy.txt. (You should create these input files by typing the information to notepad and save with the above names)
Two input files should be read parallel line by line
-For each line of each file: split information, calculate the requested values in columns then write on the screen and write to the output file named SaleComparison_yourLastName_ xxxx_and_yyyy.txt as follows:
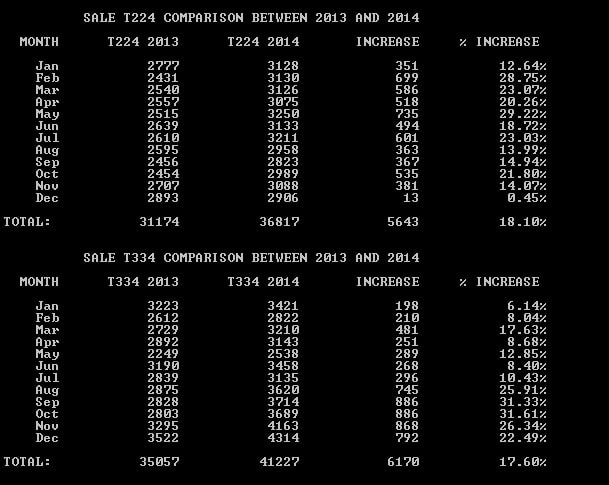
NOTES:
-split the one line of the input file into 5 information month, T224 units sold, T224 unit price, T334 units sold, T334 unit price.
For example: aLineReadFromFile = “first second third fourth fifth”
#include <sstream>
istringstream iss;
iss.str(aLineReadFromFile);
string first; iss >> first;
string second; iss >> second;
string third; iss >> third;
string fourth; iss >> fourth;
string fifth; iss >> fifth;
-convert a string to int: int number = stoi(astring);
-convert a string to double: double number = stod(astring);
-calculate the monthly sold each model by:
month_T224 = T224 units sold * T224 unit price
month_T334 = T334 units sold * T334 unit price
-calculate the sum of money sold in the year each model:
year_T224 = year_T224 + month_T224
year_T334 = year_T334 + month_T334
HOW TO DO THE PROJECT
-Review how the user-defined function works. Also, how to pass the argument by reference. For example:
{`void foo (int & x)//x is now a reference
{
x = x+1;
}
int main ()
{
int a = 2;
cout << “a = “ << a << endl; // output a = 2
foo (a); //Call user-defined void function named foo
cout << “a = “ << a << endl; // output a = 3
}
`}
-Review read input from the keyboard by cin to read the year to create the file name
-Review how to open a file with the file name to read and how to close the file. You can read the topic “Open file to read and check if existing” from Learning From Questions page on eCampus
-Review how to open a file with the file name to write and how to close the file. You can read the topic “Open file to write AND to append” from Learning From Questions page on eCampus
-Review read one line of the file by using getline(fileReference, filename)
{`-Use while (!inputFile.eof()) { read and process one line }`}
-Read the topic “Split a string with a delimeter” from the Learning From Question on eCampus to know how to split information from one line that read from the file
-Read the topic “Convert a string of digits into a int number or a float number” on eCampus to know how to convert a string that is splitted from the line to a number to calculate.
-Review how to display the information in columns with setw
-Review how to display a decimal number in 2 decimal digits with:
cout.precision(2);
cout << fixed << showpoint;
-Read the topic “Re-display the menu by using do.. while loop” from Learning From Questions page on eCampus to allow users to continue using your application until they want to exit
Create a project named SU15PROJECT_LastName then add the source file named SU15PROJECT_LastName_ SaleReport.cpp that contains main()
In the main(), do the following:
-Display the menu to allow users to select
- Summary Sale in one year
- Sale Comparison between two years
The application should allow store managers to continue using until they want to exit. That means after finishing one task the program should loop back to display the menu again until the users want to exit
-After read selected task:
TASK1: (Summary Sale in one year):
Create a method to do the following
-Asks users to enter 4 digits for the year in yyyy format, then open the input file Model_T_Sale_yyyy.txt to read
-For each line, split the information into 5 data: month, T224_Units, T224_uPrice, T334_Units and T334_uPrice.
-Calculate the following to prepare for output
- the column T224 includes the monthly sale the product T224
T224 SALE = T224 units sold * T224 unit price
-the column T334 includes the monthly sales the product T334
T334 SALE = T334 units sold * T334 unit price
TOTAL is added in columns
Total sale in year: is added total sale of two models
-Display the result in columns as requested (see output for task1 above)
TASK2: (Sale Comparison between two years):
Create a method to do the following:
-Asks user to enter 4 digits of 2 years to compare in the format “xxxx yyyy”
-Open 2 input files, Model_T_Sale_xxxx.txt and Model_T_Sale_yyyy.txt.
-We will read two input file parallel.
For each line of each file, split information into 5 data: month, T224_Units, T224_uPrice, T334_Units and T334_uPrice
*calculate the following to prepare for output:
-Column INCREASE: is calculated by: number of units sold in 2014 – number of units sold in 2013
INCREASE = T224_2014 – T224_2013 (number of units sold in 2014 – number of units sold in 2013)
-Column % INCREASE is calculated by: INCREASE / number of units sold in 2013
%INCREASE = (double) INCREASE / (double) T224_2013 (INCREASE / number of units sold in 2013)
-TOTAL:
In the second and third columns are the sum of number of units sold in 12 months the year
In the fourth column is the different of the total number of T334 units in the year and the total number
of T224 units in the year
In the fifth column is calculated as below:
% INCREASE = total of INCREASE / total of number of T224 units sold in the year (second column)
*The information of the table of SALE T334 COMPARISON is calculated as the same as for T224
*save the month, number of T224 units sold in the first year, number of T224 units sold in the second year, monthly increase and monthly % increase into 5 arrays with the same size
*save the month, number of T224 units sold in the first year, number of T224 units sold in the second year, monthly increase and monthly % increate into 5 arrays with the same size
Create a method to display the information of above arrays on the screen and also write to the file in the requested format (see the output file in the task 2 above)
HOW TO TURN IN PROJECT:
Submit on eCampus the source files:
- SU15PROJECT_LastName_ SaleReport.cpp
- SU15PROJECT_LastName.exe
- One output file Sale_Summary_yyyy.txt (output file for year yyyy of task 1)
- One output file SaleComparison_xxxx_and_yyyy.txt (output file of task 2)
- pseudo-code or flowchart of the main() in word document SU15PROJECT_LastName.docx
Submit all files requested with correct names |
2 |
turn in on time |
10 |
compile success with all the requirements |
10 |
Pseudo-code or flowchart in project_yourLastName.doc |
5 |
Write the comment in the program |
5 |
Using at least 3 user-defined functions and call them in main() |
12 |
The user defined functions using the reference parameters |
6 |
Manage main menu to re-display after finishing each task and terminate when users selects exit |
3 |
TASK1: Open file to read, open file to write, close file, while loop to read the file, read one line, split the information of one line correct, calculate month sale, calculate year sale, display the output on screen and write the output file in correct format |
9 |
Out put of task 1 is in the requested format |
3 |
TASK2: open 2 files to read, open file to write, close files, while loop to read 2 files, read one line on each file, split information of one line correct, calculate INCREASE, calculate %INCREASE, and all Totals correct |
12 |
Output of task 2 is in the requested format |
3 |
PROJECT SCORES |
80 POINTS |