Parallel image processing assignment 1
Introduction
This assignment aims to consolidate your understanding of several topics taught in this course, such as applied functional programming and parallel multicore programming. The actual application covers a small set of fundamental image processing tasks, one of which is still an active research topic (thinning).
Overview
You are required to research the required topics and then design and implement one command-line F# program, called a1.exe, with the following features:
- a1 infile options … : reads the image indicated by infile, and starts processing it as indicated by options, by default in the sequential The following file formats should be accepted: BMP, GIF, EXIF, JPG, PNG and TIFF.
- /SEQ : turns on the sequential mode for the next options (this is laos the default).
- /PAR : turns on the parallel mode for the next options.
- /STATS : transforms the input image according to the following statistics: min, mean, median, max; and saves the results as bmp, infilename_mean.bmp, infilename_median.bmp, infilename_max.bmp, respectively.
- /THIN : transforms the input image by thinning the foreground (aka skeletonization), using the Guo-Hall algorithm – please do a bit of research on this! The result is saved as bmp.
- /THRESHOLD t : sets the gray to b/w transformation threshold for the next options; t must be an int between 0 and 255, by default 200.
- /BFG and /WFG : switch between the blackforeground and the whiteforeground for the next options; this convention is necessary for the THIN transform; the default is black
All transformations must use the 8-neighborhood, i.e. the current pixel plus its NW, N, NE, E, SE, S, SW, W neighbours (where these exist, attention on border cases).
The STATS transforms must be performed in a gray scale; the image must be converted, if necessary, according to the following formula:
let gray (c:Color) = ((int c.R) + (int c.G) + (int c.B)) / 3
The THIN transform must be performed on a b/w scale; the image must be converted, if necessary, according to the following pixel transformation:
(fun x -> if x < THRESHOLD then 0 else 255)
The THIN transform needs to know which colour indicates the foreground to be thinned; the default is black.
Each processing step must be timed and the timing must be printed on the console; the timing must include all needed transformations (including conversions to/from the gray or b/w scale), with the exception of the actual reading and writing (but no more than this).
Development tools
If you wish, you can first develop your solution using VS 2012 or Linqpad, but, in the end, please reshape your solution as a set of command-line compilable files.
You can use any of the existing standard .NET and F# libraries, but NO other third party library or source (thus, no OpenCV, please).
Assessment
Each solution will be independently assessed on (1) correctness; (2) readability; (3) functional style; (4) abstraction level; (5) performance.
The correctness includes (a) following the specs; (b) using standard libraries only; (c) obtaining the expected results.
We expect that the parallel versions share much of their code with their sequential counterparts.
We generally expect that both the sequential and parallel versions run in reasonable time and that the parallel versions are faster than the sequential ones, on a typical lab machine.
The report is expected to be short (4-6 pages) and should include: (1) a short discussion and a citation to the article(s) or page(s) used for your skeletonization implementation;
(2) an assessment of your own results (could include tables and plots); (3) conclusions; (4) short bibliography. The report should be written in the LNCS article style (LaTeX or Word).
Deliverables and submission
Submit electronically, to the new COMPSCI web dropbox, an upi.7z archive containing an upi folder with:
o your report as PDF; o your F# source file(s); o a batch file to compile your file(s) into an executable and run a few sample tests.
Please keep your electronic dropbox receipt and follow the instructions given in our Assignments web page (please read these carefully, including our policy on plagiarism):
http://www.cs.auckland.ac.nz/courses/compsci734s1c/assignments/
Appendix 1 - Samples
/STATS – images of gray scale memory arrays backing the actual images
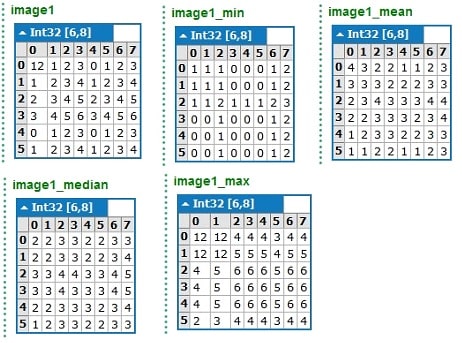
/WFG /THIN
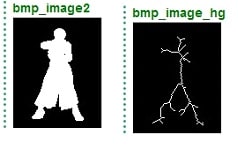
/BFG /THIN
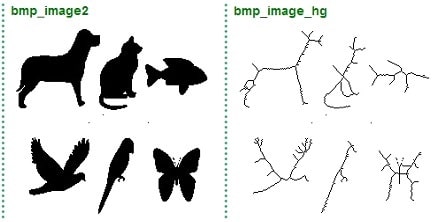