Javascript Project 3
This semester we have learned the basics of JavaScript as well as how to use the jQuery library to make accessing HTML elements easier so that we can create better user experiences. In this project, you will get to use
Project Goal
A local company needs you to create a solution that will allow the user to enter a username and a password into a form, and hit a submit button. The real database interaction will happen with future server side coding done by someone else. But for now, your job is to simulate the login process as described below.
Project Notes
The company currently uses another javascript library named Bootstrap. Bootstrap is a very popular framework that basically uses jQuery to create responsive pages. It was developed by twitter developers and includes a js file as well as a css file that aims at creating consistent page elements. So that is included in your file. This is nothing you have to learn, but if you want to take a look at it, please do so. So ignore the menu on the page and just focus on the form itself.
Assessment
This program assess the student’s understanding of
- Variables
- Operators
- Functions
- Selectors
Requirements
Create a new file named login.js and save it inside your js folder for this project. This is where all your js code should be placed.
- When the user enters an email address and password, and then hits submit,
- First make sure you stop the form from submitting, and then,
- create and call a function that does the following:
- First checks to see if a username was entered.
- If it does not include the above, then change the text in the “message” id element to read an appropriate message and return false.
- Also add a class named “error” to the paragraph so it changes the text to show red bold font.
- Else move on to the password
- Check to make sure it has a password entered
- If not, change the text in the “message” id element to read an appropriate message and return false.
- Also add a class named “error” to the paragraph so it changes the text to show red bold font.
- If anything at all is entered for both the username and password, then return true and:
- Remove the “error” class from the paragraph
Now before you move on, save and test this to make sure it is working. Test by doing this:
- Without entering anything into the text boxes, hit submit
- If it is working correctly, it should change the text in the top of the form to show your error in red bold font.
- Add something to the email field and then hit submit. It should show you an error for the password box similar to above.
- If all this is not working, do not move ahead. Troubleshoot this and get it working.
Now let’s do a check on the actual values entered into the text boxes to make sure they match the hard coded values I left for you at the top of the index page. We are simulating that we are checking a database for those values. So if they match, let them in. If not, let them know.
- Create another function that is called if the first function returns true, and:
- Compare the username entered in the form to the variable value found in your index page at the top.
- If it matches, then continue on and check the password.
- If either is not the correct value, then return a message similar to the previous messages, telling them which value is not correct.
- Remember to add the “error” class if there is an error message. iv. Or remove it if it returns true.
- If everything checks out, do the following:
- Also add a class named “success” to the paragraph so it changes the text to show green bold font.
- Change the paragraph text to let them know they successfully logged in.
Now before you move on, save and test this to make sure it is working. Test by doing this:
- Enter an incorrect email address into the username field and something into the password field Hit enter, it should return an error message if they do not match.
- Test it the same way using the wrong password and make sure the correct message shows up
If all that works, then move on to this last step:
Since we are doing all of this on a page that does not refresh at all, we need a way to let the user know that something is happening when he/she hits the submit button. This can be done in a number of ways, but a common feature is to show a loading type image while the process is taking place, and then stop it from showing when the process returns.
Note: since we are not really hitting a server here, we are going to add a new function that will simulate it taking a few seconds to execute.
So do the following:
- Add this function around the calls to the custom functions you created:
setTimeout(function() { }, 3000);
- Example, I created a function named checkUsernamePassword() and if that returned true, I called another function that compared the username and password data entered. So my code would look like this: setTimeout(function() {`{`} if(checkUsernamePassword())
{`{
if(compareUsernamePassword())
{
//show welcome message if they logged in successfully
}
}
}, 3000);
`}
What this does is sets a time frame of 3 seconds before anything within that function starts to execute.
Test it now to make sure you did it correctly:
- Hit the enter button and wait 3 seconds, and it should show you an error.
- If it does not, then you need to check your code as you may have set it up different than mine so you just need to figure out where to add that setTimeout function.
- Now lets call the spinner.
You will notice there is a spin.js file included at the bottom of your index page. This is a pretty cool script that uses code to create the spinner instead of using an animated image that takes up file size. In order to call it, just go to your submit function and add these two lines at the top of the function (inside it), but not within the setTimeout() function.
var target = document.getElementById('loginform'); var spinner = new Spinner(opts).spin(target);
Save and refresh your page, then hit the submit button, you should see a spinner in the center of your login box. If not, look at the errors and troubleshoot your page. If it works, move on to the last few lines you need this semester!!
He only thing left now is to actually stop the spinner from showing once we are done. So to do that, this particular script has a built in method you can call that will stop it.
- Add the following line just above the closing part of the setTimeout function so it looks like this:
spinner.stop();
{`}, 2000);`}
It should now stop when the message changes if there are any errors, or when the timeout completes otherwise.
Walk through and test everything again to make sure you got it all. JavaScript is all about solving problems, so think about the errors you are getting and try to figure out how to solve them.
Review everything again and make sure you did not miss one of the requirements.
When you are done with this assignment, please do the following:
- Connect to FTP
- Then move your “login” folder over to the server inside your secret folder.
- Verify your file is there by browsing to your assignment index file on the server by clicking on the links for Project # 3 and making sure it shows up.
Grading Rubric for this Project | |
1. All code was commented completely so I could follow along with your logic |
10 points |
2. Function created that checked to make sure there was a value entered inside both fields, and returned proper message. |
30 points |
3. Function created that compared both the username entered and the password entered with the hard coded values found in the variables. Also returned proper message. |
30 points |
4. setTimeout function added correctly, with spinner starting and stopping as required. |
30 points |
Total: |
100 points |
Here is how the page flow should go (more or less):
If the form has not been submitted yet, it should look like this:
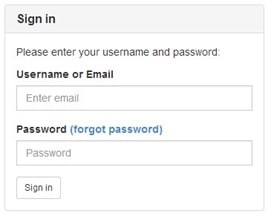
If the form was submitted, but left blank, it should look like this (depending on which field is blank):
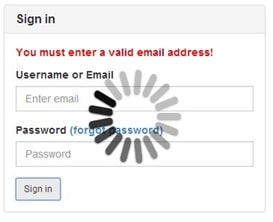
If the form was submitted, both fields had data entered, but incorrect email address, it should look like:
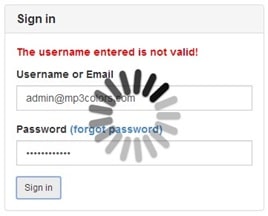
If the username and password both match, it should look like:
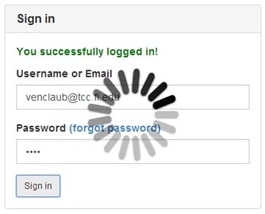