how to write menu in python
Free menu python code.
import gps
def print_menu ():
print "MAIN MENU"
print "A - Reset GPS"
print "B - Update Current Location"
print "C - Save most recent update as Waypoint"
print "D - Add Waypoint to Path"
print "E - Remove Waypoint from Path"
print "F - Distance between waypoints"
print "G - Total Path Length"
print "H - Distance from current location to waypoint"
print "I - List all stored waypoints by name"
print "J - List all stored Waypoints by name and coordinates"
print "K - List all stored Paths"
print "L - List Waypoints on Path"
print "X - Exit"
var = raw_input("Enter selection by letter --> ");
return var;
g = gps.GPS()
while 1:
option = print_menu()
if (option == "A" or option == "a"): g = gps.GPS()
elif (option == "B" or option == "b"):
p = g.gpsUpdateCurrentLocation()
print "Long: ", p.longitude, " Lat: ", p.latitude
elif (option == "C" or option == "c"):
name = raw_input("Enter Waypoint name: ")
g.gpsSaveWaypoint(name)
elif (option == "D" or option == "d"):
print "Select Waypoint to add:"
g.gpsListAllWaypoints()
wp_name = raw_input("Waypoint to add: ")
print "Path defined so far:"
g.gpsListAllPathNames()
path_name = raw_input("Path to add to (or new name for new path):")
g.gpsAddWaypointToPath(path_name, wp_name)
elif (option == "E" or option == "e"):
print "Select Waypoint to remove:"
g.gpsListAllWaypoints()
wp_name = raw_input("Waypoint to add: ")
print "Path defined so far:"
g.gpsListAllPathNames()
path_name = raw_input("Path to remove from:")
g.gpsRemoveWaypointFromPath(path_name, wp_name)
elif (option == "F" or option == "f"):
print "Waypoints known:"
g.gpsListAllWaypointNames()
name1 = raw_input("First waypoint name: ")
name2 = raw_input("Second waypoint name: ")
dist = g.gpsComputeDistance(name1, name2)
print dist, " km"
elif (option == "G" or option == "g"):
print "Paths known:"
g.gpsListAllPathNames()
name = raw_input("Path: ")
length = g.gpsComputePathLength(name)
print "Total path length is ", length, " km"
elif (option == "H" or option == "h"):
print "distance from waypoint"
elif (option == "I" or option == "i"):
g.gpsListAllWaypointNames()
elif (option == "J" or option == "j"):
g.gpsListAllWaypoints()
elif (option == "K" or option == "k"):
g.gpsListAllPathNames()
elif (option == "L" or option == "l"):
g.gpsListAllPathNames()
name = raw_input("Select path: ")
g.gpsListPath(name)
elif (option == "X" or option == "x"): break
else: print "Invalid Choice"
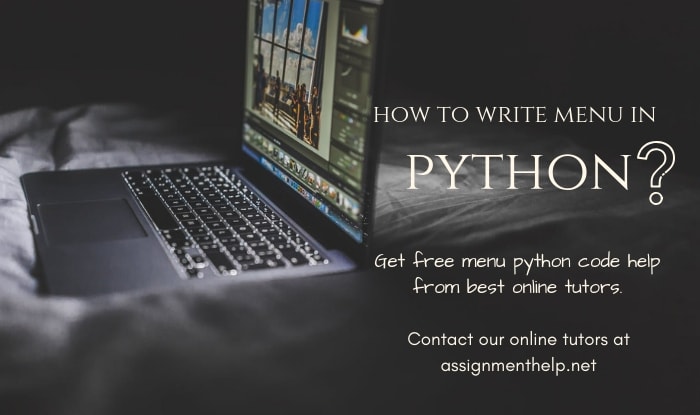
- HTML And Python
- Variables
- Comments
- Operators
- Controls And Loops
- Functions
- Events
- Error And Exceptions Handling
- Functions
- String
- Array
- Stacks
- Queues
- Lists
- RegExp
- Dictionaries
- Graphs
- Input And Output
- Modules
- Classes
- Handling Cookies
- Browser And Session
- Validation
- Date And Time
- Data Compression
- String Pattern Matching
- File Handling