How to make Calculator in C#?
Algorithms: -
Design Parts
- Take Windows Form App (.net Framework)
- Select Button tool from Toolbars and Drag it on Window Form App.
- Copy it and Paste 17 times on Blank Window Form App.
- Rename the Button Text as 0, 1,2,3,4,5,6,7,8,9 and (.)
- Go to properties of each Button and change the Forecolour and backcolor.
- Rename 4 Button As operator sign (+, -, *, /)
- Rename rest of Button as C, CE And =
- Now click on any Button option between 0 to 9 and Go to event option in Properties, Choose Click option and Rename it as button_click. Copy the Same text and paste it on rest Button of Numeric value.
- Now Click on any operator Button, go to event option in properties, choose click option and rename it as operator click. Copy the same text and paste it on rest of operator Button on Click event.
- Select Text tool from Toolbars and drag it on Window Form App.
- Change the Properties like Forecolour, Text Align and Backcolor as per your choice.
- Select Label from Toolbars and Drag it on Window Form App and Set properties.
- In Label box the operation will display with output.
- Click any where in Window Form App, go to properties and Name TEXT as Simple Calculator.
- Now double-click on any numeric Button Form1.cs will open.
Programming Parts
1. Define the variable in the class. Below is the code.
{` public partial class Form1: Form { Double resultvalue = 0; String operationPerformed = ""; bool isOprationPerformed = false; public Form1() { InitializeComponent(); }`}
2. On double click on any numeric Button, Form1.cs will open and Cursor will blink near Numeric Button. Paste the below code between open and close bracket.
{` private void button_click(object sender, EventArgs e) { if ((textBox_Result.Text == "0") || (isOprationPerformed)) textBox_Result.Clear(); isOprationPerformed = false; Button button = (Button)sender; if (button.Text == ".") { if (!textBox_Result.Text.Contains(".")) textBox_Result.Text = textBox_Result.Text + button.Text; }else textBox_Result.Text = textBox_Result.Text + button.Text; }`}
3. Now Double click on any operator button it will again open the Form1.cs and cursor will near operator button. Paste the below code between open and close bracket.
{` private void operator_click(object sender, EventArgs e) { Button button = (Button)sender; if (resultvalue != 0) { button15.PerformClick(); operationPerformed = button.Text; label_CurrentOperation.Text = resultvalue + " " + operationPerformed; isOprationPerformed = true; } else { operationPerformed = button.Text; resultvalue = Double.Parse(textBox_Result.Text); label_CurrentOperation.Text = resultvalue + " " + operationPerformed; isOprationPerformed = true; } }`}
4. Now double click on CE Button, Form1.cs Will open and Paste below code
{` private void button5_Click(object sender, EventArgs e) { textBox_Result.Text = "0"; }`}
5. Again double click on C Button And paste the below code.
{` private void button10_Click(object sender, EventArgs e) { textBox_Result.Text = "0"; resultvalue = 0; }`}
6. Now double click on Equal to (=) Button and Paste the below code will will perform the operation.
{` private void button15_Click(object sender, EventArgs e) { switch(operationPerformed) { case "+": textBox_Result.Text = (resultvalue + Double.Parse(textBox_Result.Text)).ToString(); break; case "-": textBox_Result.Text = (resultvalue - Double.Parse(textBox_Result.Text)).ToString(); break; case "*": textBox_Result.Text = (resultvalue * Double.Parse(textBox_Result.Text)).ToString(); break; case "/": textBox_Result.Text = (resultvalue / Double.Parse(textBox_Result.Text)).ToString(); break; default: break; } resultvalue = Double.Parse(textBox_Result.Text); label_CurrentOperation.Text = ""; }`}
7. Now Debug the code.
NOTE:- private void button15_Click(object sender, EventArgs e) is inbuilt function when double click on Button. So don’t write it again in the program.
OUTPUT
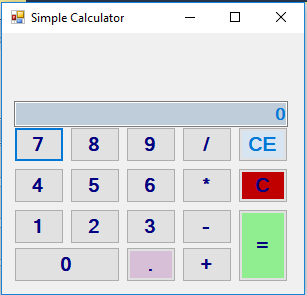