Windows PowerShell AssignmentHelp
Windows PowerShell is Microsoft's task automation framework, consisting of a command-line shell and associated scripting language built on top of and integrated with the .NET Framework. PowerShell provides full access to COM and WMI, enabling administrators to perform administrative tasks on both local and remote Windows systems.
We present Window PowerShell programming help from basic to higher level. Our expert programmers are always available to provide help. Online tutorial service is also available for everyone who wishes to learn the programming language.
What Assignment Help for Window power shell programming language we provide?
All type of the Assignment Help are provided here. Students who are facing problem with the Window PowerShell then they can visit assignmenthelp.net and get solution by the means of chatting with our expert. Student can also join online tutorial and learn the Window PowerShell programming language in very effective manner at a very nominal cost in very less time.
Windows PowerShell Introduction
Windows PowerShell is a powerful command-line shell and scripting language developed by Microsoft. It is designed to help system administrators and power users automate and manage tasks in the Windows operating system. PowerShell provides a wide range of functionalities for managing and configuring Windows systems, as well as interacting with various software and services.
Here is a basic introduction to Windows PowerShell:
-
Interactive Shell: PowerShell provides an interactive command-line interface where you can enter commands and receive immediate feedback. This is often referred to as the "PowerShell console" or "PowerShell prompt."
-
Scripting Language: PowerShell is not just a command-line interface; it is also a scripting language. You can write scripts (sequences of PowerShell commands) to automate tasks, create custom functions, and perform complex operations.
-
Object-Oriented: PowerShell is object-oriented, which means it deals with objects rather than just text. Many commands return objects that you can manipulate and pass to other commands, making it more versatile and powerful than traditional command-line interfaces.
-
Cmdlets: PowerShell commands are called "cmdlets" (pronounced "command-lets"). Cmdlets are specialized .NET classes designed to perform specific tasks. They follow a verb-noun naming convention (e.g.,
Get-Process
,New-Item
,Remove-Item
), which makes it easy to understand their purpose. -
Pipeline: One of the most powerful features of PowerShell is the pipeline, which allows you to chain multiple cmdlets together. You can take the output of one cmdlet and use it as input for another, making complex operations more straightforward.
-
Script Execution Policy: PowerShell has a security feature called "script execution policy" that determines whether and how scripts can be run. It can be set to allow or restrict script execution to protect against potentially malicious scripts.
-
Modules: PowerShell can be extended using modules. Modules are collections of cmdlets, functions, and scripts that can be imported into your PowerShell session to extend its capabilities.
-
Integration: PowerShell can interact with various Microsoft technologies, such as Active Directory, SQL Server, Exchange Server, and more. It also supports REST API calls, making it versatile for managing a wide range of resources.
-
Cross-Platform: PowerShell is not limited to Windows. With the introduction of PowerShell Core (now known as PowerShell 7 and later), it became cross-platform, allowing you to use it on macOS and Linux in addition to Windows.
-
Script Files: PowerShell scripts are saved in .ps1 files. You can create, edit, and run these script files to automate repetitive tasks or perform complex operations.
To get started with Windows PowerShell, you can open a PowerShell console by searching for "PowerShell" in the Start menu or by pressing Windows Key + X
and selecting "Windows Terminal (PowerShell)." From there, you can start entering commands, exploring cmdlets, and learning how to automate tasks.
Windows PowerShell Assignment Help By Online Tutoring and Guide Sessions at AssignmentHelp.Net
Brief introduction and proper documentation are available for all students from college and school. All help related to the assignment, project, homework and programming language is available on assignmenthelp.net. Our services are open for all at normal cost.
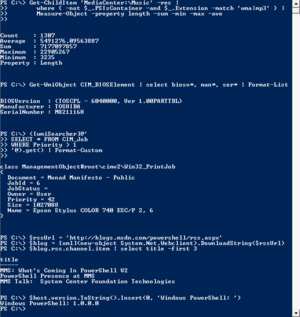
Features of Window power shell programming language:
- Desired State Configuration
- New Default Execution Policy
- Enhanced debugging:
- Pipeline Variable switch:
Window power shell programming Code
Hello World in PowerShell:
{` "Hello, world!" Write-Host "Hello, world!" echo "Hello, world!" [System.Console] :: WriteLine("Hello, world!") `}
Variables:
$myVariable = "This is a variable" Write-Host $myVariable
User Input:
$yourName = Read-Host "What's your name?" Write-Host "Hello, $yourName!"
Conditional Statements:
$number = 42 if ($number -eq 42) { Write-Host "The answer to everything is $number." } else { Write-Host "The answer to everything is a mystery." }
Loops:
# For Loop for ($i = 1; $i -le 5; $i++) { Write-Host "Iteration $i" } # While Loop $i = 1 while ($i -le 5) { Write-Host "Iteration $i" $i++ }
Functions:
function SayHello { param( [string]$name ) Write-Host "Hello, $name!" } SayHello -name "Alice"
Working with Files:
# Read a text file $content = Get-Content "C:\path\to\file.txt" Write-Host "File content: $content" # Write to a text file Set-Content "C:\path\to\output.txt" "This is some text."
Working with Processes:
# List running processes Get-Process # Start a new process Start-Process -FilePath "notepad.exe" # Stop a process by name Stop-Process -Name "processName"
Working with Services:
# List all services Get-Service # Start a service Start-Service -Name "serviceName" # Stop a service Stop-Service -Name "serviceName"
Error Handling:
try { # Code that might generate an error $result = 10 / 0 } catch { # Handle the error Write-Host "An error occurred: $_.Exception.Message" } finally { # Code that will always run Write-Host "Finally block executed." }
These are just some basic examples to get you started with PowerShell.
List of popular Programming Languages and its tutorials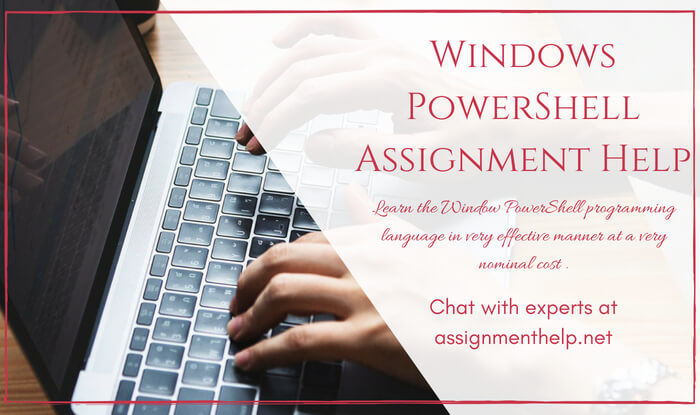