Write a C# program that allows you to produce a table of values
Write a program that allows you to produce a table of Football or Hockey teams. The table should also include games played and points. Attempt this program with some argument passing. For higher grades attempt below procedure Improve the array by sorting it by points. For example, the team with the highest points should be at index 0.
Procedure:
- Go to File, New -> Project, select window Classic Desktop ->Console App (.net Framework).
- Console App created.
- Create a table and mention the column name in the table.
- Insert the value in the table.
- Sort value in DESC order by point.
- Code is given below.
C Sharp Program Code:
{` using System; using System.Data; className Program &lbrace static void Main() &lbrace string Player; using (DataTable table = new DataTable()) &lbrace table.Columns.Add("Player", typeof(string)); table.Columns.Add("Game", typeof(string)); table.Columns.Add("Points", typeof(int)); for(int i = 0; i < 3 ; i++) &lbrace Console.WriteLine("Enter the " + (i+1) + " player Information"); Player = Console.ReadLine(); string[] abc = Player.Split(' '); int score = Convert.ToInt32(abc[2]); table.Rows.Add(abc[0], abc[1], score); &rbrace DataView view = new DataView(table); view.Sort = "Points DESC"; Console.WriteLine("\nRows in sorted order\n \n"); foreach (DataRowView row in view) &lbrace Console.WriteLine(" {0} \t {1} \t {2} ", row["Player"], row["Game"], row["Points"]); &rbrace &rbrace &rbrace &rbrace `}
C Sharp Program Output:
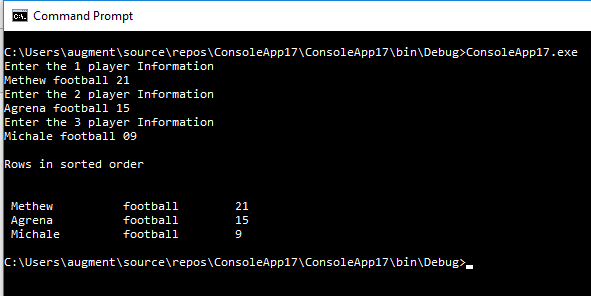