Hangman game Assignment Help
Game concept: the user should guess random word selected by computer from the command prompt. When the user starts the game from the command prompt. The user gets prompt to choose the difficulty of the game: easy, medium or hard. If user enters easy a random word will be selected from easyWordsList by computer:
Example:
{` private String[] easyWordsList = {"ant", "bat"}; private String[] mediumWordsList = {"field", "array"}; private String[] hardWordsList = {"thursday", "language"}; private String[] allWordsList = {"ant","bat","field","array","language","thursday"}; and _ _ _ equal to the number of letters in word displays on the screen `}
If the user doesn't enter any difficulty and clicks enter button, a random word will be selected from allWordsList
Hangman game Assignment Help By Online Tutoring and Guided Sessions at AssignmentHelp.Net
The game starts and User should guess the correct word now
{` If user guesses correct letter, message guess next letter displays *if user guesses the wrong letter, message incorrect letter guessed displays and different letter displays *if guesses all letters, message you won game displays *if fails to guess, within given number of tries, sorry you lost game message displays *user should be able to quit game anytime by typing “quit” *game is not case sensitive i.e., ANT is same as ant *if user enters numbers, message numbers are not allowed displays *user guessed letters always displays *number of tries user can guess is 5. When user guesses wrong character, tries will decrease by 1. If tries =0 user will lose game `}
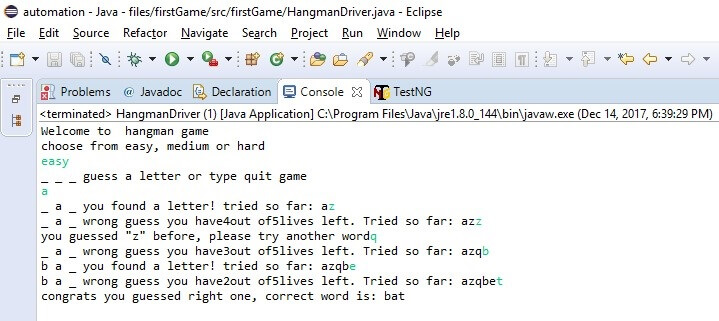
{` *Write hangman game using two classes: 1. HangmanDriver className AND 2. Hangman className *HangmanDriver className contains main method to run game (see sample starter code) *Hangman className contains : use enum to select difficulty (easy, medium and hard), method to set difficulty, method to randomly pick word from word list, method to run game and other methods as required Starter Code for Hangman.java pubic className Hangman { // variables // counters, word arrays, etc constructor with param public Hangman(Difficulty difficulty) { setDifficulty(diffculty); } // default constructor pubic Hangman() { // select a difficulty randomly setDifficulty(randomDiffculty); } // function to run the game public void runGame() { // prompt user input (this can be done in HangmanDriver.className if desired) // set difficulty // pick word // prompt user input // validate and check if match found // display result, attempts, and letters and word tried // repeat until user runs out of life, guesses the word, or types "quit game" } // method that sets difficulty of the game // method that picks a word // method that prompt the user // method that use validates user input // method that checks for matches // method that displays the game status // more methods as necessary } Starter code for HangmanDriver.java public className HandmanDriver { public static void main(String[] args) { Hangman hangman = new Hangman(); hangman.runGame(); } /* OR public static void main(String[] args) { // prompt user input for difficulty // if empty new Hangman() // else new Hangman(difficulty) // runGame } */ } `}