Stacks Implementation Assignment Help
A "stack" is a fundamental data structure in computer science that follows the Last-In-First-Out (LIFO) principle. It's like a collection of items where you can add or remove elements only from the top, much like a stack of plates. The last item added is the first one to be removed. Stacks are used in various algorithms and programming scenarios, such as parsing expressions, managing function calls in a program, and implementing features like the back button in web browsers.
An implementation of a stack involves creating the necessary operations to manipulate the stack, typically including:
-
Push: This operation adds an element to the top of the stack.
-
Pop: This operation removes and returns the top element of the stack.
-
Peek (or Top): This operation returns the top element of the stack without removing it.
-
IsEmpty: This operation checks whether the stack is empty or not.
-
Size: This operation returns the number of elements currently in the stack.
You can implement a stack using various programming languages, and the choice of implementation may vary depending on the language's features and the specific requirements of your application. Common implementations include using arrays, linked lists, or dynamic arrays (like Python's list).
Here's a simple example of a stack implemented using a Python list:
class Stack: def __init__(self): self.items = [] def push(self, item): self.items.append(item) def pop(self): if not self.is_empty(): return self.items.pop() def peek(self): if not self.is_empty(): return self.items[-1] def is_empty(self): return len(self.items) == 0 def size(self): return len(self.items)
In this example, the Stack
class uses a list (self.items
) to hold the elements of the stack. The methods push
, pop
, peek
, is_empty
, and size
provide the basic operations for working with the stack.
The Stack Implementated As An Array
One of two ways to implement a stack is by using a one dimensional array (also famed as a vector). When implemented this way, the data is simply stored in the array. Top is an integer appreciate, which contains the array index for the top of the stack. Each time data is additional or removed, top is incremented or decremented accordingly, to keep track of the current top of the stack. By convention, an empty stack is indicated by setting top to be equal to -1.

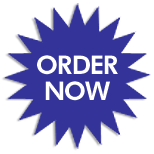
Find the best Stacks Assignment Help Services with us
Try our determination care now, solution of your problem is righteous a depression departed. Knock any quantify at our 24x7 live supports for any ask. To know about how to proceed, just visit how it Works page at Assignmenthelp.net.
Stacks Assignment Help | Stacks Homework Help | Stacks Online Tutoring | DBMS Assignment Help | Database Design | Online Tutor | SQL Server Database | Database System | Assignment Help | Relational Database | Database Management System | Schema Database Homework Help | MS Database | Database Application Assignment Help | XML Database Homework Assignment Help | Object Database | Database Object Oriented | Introduction To Database Homework Help | Dissertation Help | Term Paper Help